Mutual TLS (mTLS) authentication creates a secure framework for authenticating both users and IoT devices. This guide will walk you through implementing mTLS to ensure secure bidirectional authentication between clients and servers.
Quick Start Guide
Setting up mutual TLS follows a straightforward process. First, generate the necessary server and client certificates. Next, configure your server to require client certificates. Then set up your clients with their certificates and implement proper certificate validation. Finally, test the connection to ensure everything works as expected.
One-Way and Mutual SSL/TLS Authentication
One of the defining features of the SSL/TLS protocol is its role in authenticating otherwise anonymous parties on computer networks (such as the internet). When you visit a website with a publicly trusted SSL/TLS certificate, your browser can verify that the website owner has successfully demonstrated control over that domain name to a trusted third-party certificate authority (CA), such as SSL.com. If this verification fails, then the web browser will warn you not to trust that site. For most applications, SSL/TLS uses this sort of one-way authentication of a server to a client; an anonymous client (the web browser) negotiates an encrypted session with a web server, which presents a publicly trusted SSL/TLS certificate to identify itself during the SSL/TLS handshake: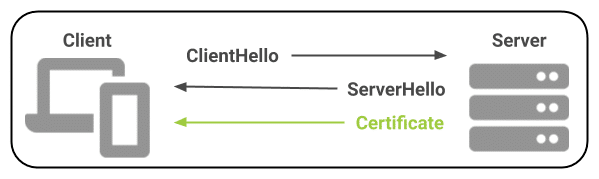
CertificateRequest
message to the client. The client will respond by sending a certificate to the server for authentication:
Client Authentication (1.3.6.1.5.5.7.3.2)
Extended Key Usage (EKU) is installed on the client device. All of SSL.com’s Email, Client, and Document Signing certificates include client authentication.
Detailed Implementation Guide
Understanding Mutual TLS
Traditional TLS provides server authentication and encryption, but mutual TLS goes further by requiring both parties to present digital certificates. This two-way verification ensures server authenticity, enables client authentication, establishes an encrypted communication channel, and prevents man-in-the-middle attacks. The result is a highly secure connection suitable for sensitive applications and IoT device communication.
Prerequisites
Before beginning the implementation, ensure you have access to OpenSSL or a similar certificate management tool. Your web server must support TLS, and you’ll need either access to a Certificate Authority (CA) or the ability to create a private CA. Your clients must also support certificate-based authentication.
Step 1: Certificate Generation and Management
Start by generating the necessary certificates for both server and client authentication. The server certificate setup requires creating a private key, generating a certificate signing request, and signing the certificate with your CA.
# Generate server private key
openssl genrsa -out server.key 2048
?
# Create server certificate signing request (CSR)
openssl req -new -key server.key -out server.csr
?
# Sign the server certificate with your CA
openssl x509 -req -in server.csr -CA ca.crt -CAkey ca.key -CAcreateserial -out server.crt
For client certificates, follow a similar process to create their unique credentials:
# Generate client private key
openssl genrsa -out client.key 2048
?
# Create client CSR
openssl req -new -key client.key -out client.csr
?
# Sign the client certificate
openssl x509 -req -in client.csr -CA ca.crt -CAkey ca.key -CAcreateserial -out client.crt
Step 2: Server Configuration
The server must be configured to require and validate client certificates. Here’s an example configuration for Nginx:
server {
listen 443 ssl;
server_name example.com;
?
ssl_certificate /path/to/server.crt;
ssl_certificate_key /path/to/server.key;
ssl_client_certificate /path/to/ca.crt;
ssl_verify_client on;
ssl_protocols TLSv1.2 TLSv1.3;
ssl_ciphers HIGH:!aNULL:!MD5;
ssl_prefer_server_ciphers on;
}
For Apache servers, use this configuration:
<VirtualHost *:443>
ServerName example.com
SSLEngine on
SSLCertificateFile /path/to/server.crt
SSLCertificateKeyFile /path/to/server.key
SSLCACertificateFile /path/to/ca.crt
SSLVerifyClient require
SSLVerifyDepth 1
</VirtualHost>
Step 3: Client Implementation
Browser-based authentication requires importing the client certificate into your browser’s certificate store. Each browser handles this process differently, but generally, you’ll find the option in the security or privacy settings.
For IoT devices, you’ll need to implement certificate-based authentication in your code. Here’s an example using Python’s requests library:
import requests
?
client_cert = ('client.crt', 'client.key')
ca_cert = 'ca.crt'
?
response = requests.get('https://example.com',
cert=client_cert,
verify=ca_cert)
Step 4: Certificate Validation
Proper certificate validation is crucial for security. Your implementation should verify the certificate chain integrity, check expiration dates, validate revocation status, and ensure proper key usage. Here’s an example implementation:
from cryptography import x509
from cryptography.hazmat.backends import default_backend
?
def validate_certificate(cert_path):
with open(cert_path, 'rb') as cert_file:
cert_data = cert_file.read()
cert = x509.load_pem_x509_certificate(cert_data, default_backend())
if cert.not_valid_after < datetime.datetime.now():
raise ValueError("Certificate has expired")
try:
key_usage = cert.extensions.get_extension_for_class(x509.KeyUsage)
if not key_usage.value.digital_signature:
raise ValueError("Certificate not valid for digital signature")
except x509.extensions.ExtensionNotFound:
raise ValueError("Required key usage extension not found")
Mutual Authentication Use Cases
Mutual TLS authentication can be used both to authenticate end-users and for mutual authentication of devices on a computer network.User Authentication
Business and other organizations can distribute digital client certificates to end-users such as employees, contractors, and customers. These client certificates can be used as an authentication factor for access to corporate resources such as Wi-Fi, VPNs, and web applications. When used instead of (or in addition to) traditional username/password credentials, mutual TLS offers several security advantages:- Mutual TLS authentication is not vulnerable to credential theft via tactics such as phishing. Verizon’s 2020 Data Breach Investigations Report indicates that almost a quarter (22%) of data breaches are due to phishing. Phishing campaigns are out for easily harvested credentials like website login passwords, not the private keys to users’ client certificates. As a further defense against phishing, all of SSL.com’s Email, Client, and Document Signing certificates include publicly trusted S/MIME for signed and encrypted email.
- Mutual TLS authentication cannot be compromised by poor password hygiene or brute force attacks on passwords. You can require that users create strong passwords, but how do you know they don’t use that same “secure” password on 50 different websites, or have it written on a sticky note? A 2019 Google survey indicates that 52% of users reuse passwords for multiple accounts, and 13% of users reuse the same password for all of their accounts.
- Client certificates offer a clear chain of trust, and can be managed centrally. With mutual TLS, verification of which certificate authority (CA) issued a user’s credentials is baked directly into the authentication process. SSL.com’s online management tools, SWS API, and access to standard protocols like SCEP make issuing, renewing, and revoking these credentials a snap!
- Individuals or organizations requiring only one or a few certificates may order Email, Client, and Document Signing certificates á la carte from SSL.com.
- Protocols like SCEP, EST, and CMP can be used to automate client certificate enrollment and renewal for company-owned and BYO devices.
- For customers requiring a large quantity of certificates, wholesale discounts are available through our Reseller and Volume Purchasing Program.
Security Best Practices
Strong security requires more than just implementing mTLS:
- Implement automated certificate rotation to ensure certificates are regularly updated
- Maintain a certificate revocation list to quickly invalidate compromised certificates
- Use strong key sizes of at least 2048 bits for RSA keys
- Configure your servers to only accept secure TLS versions and strong cipher suites
Your client security strategy should include protecting private keys with strong access controls. Regular security audits will help maintain the system’s integrity over time.
Troubleshooting Common Issues
Certificate Chain Issues
When implementing mTLS, you may encounter certificate chain issues. These typically stem from:
- Incomplete certificate chains
- Improperly installed intermediate certificates
To resolve these issues:
- Verify that your trust anchor configuration is correct
- Ensure all necessary certificates are present
Connection Problems
Connection problems often relate to:
- Firewall settings blocking TLS traffic
- Incorrect certificate permissions
To troubleshoot:
- Check that your certificates are properly named
- Ensure they are matched to their intended use
Performance Considerations
Performance considerations become important at scale. To optimize performance:
- Implement session caching to reduce the overhead of repeated handshakes
- Choose efficient cipher suites that balance security and performance
- Monitor your certificate validation process to ensure it’s not creating unnecessary overhead
Conclusion